NextJS API - Temporarily Store File From URL
With NextJS apps there have been countless times when I need to upload a file to some other service and have to hold it locally.
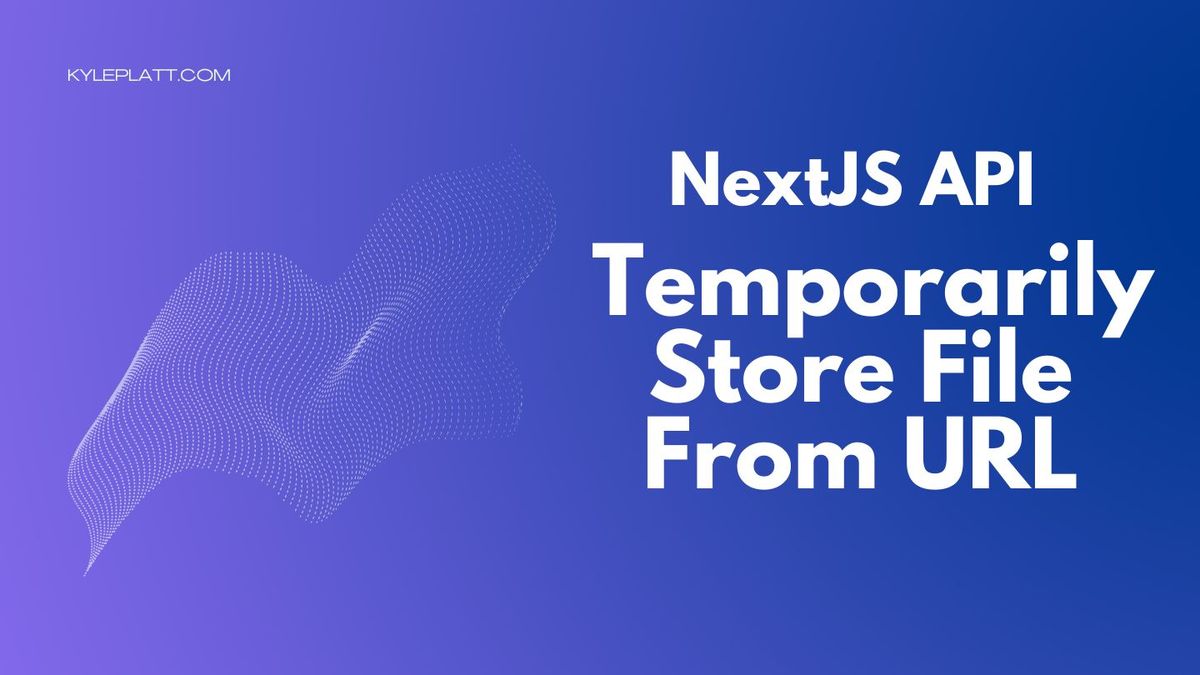
When dealing with integrations in my NextJS apps there have been countless times when I need to upload a file to some other service. The actual code to do this is very straightforward but finding the right answers was quite challenging because of some constraints:
- NodeJs - When working in the NextJS API layer you are working with NodeJs. The file handling is not the same as on the client side and you must use the 'fs' package.
- Serverless - NextJs API functions are serverless. This means you don't have the usual file management control that you would on your own server.
The Code
import { extname } from 'path';
import { writeFileSync } from 'fs';
export async function storeFileLocally(url: string): Promise<string> {
const response = await fetch(url);
const buffer = await response.arrayBuffer();
const data = Buffer.from(buffer);
const path = `/tmp/${randomString(16)}.${getFileExtension(url)}`;
writeFileSync(path, data);
return path;
}
function getFileExtension(url: string): string {
return extname(url).slice(1);
}
All the code is doing here is using fetch to grab a file by its URL and putting it into an arraybuffer. Then using writeFileSync we can write that arraybuffer to a file. The key thing to point out is that we are storing it in the /tmp folder which seems to be a preference of Vercel and a smart thing to do. Since we are working in a serverless environment we can't assume the file will be there after execution, if you need persistence then you will have to connect it to a storage service such as S3.